引言
空间查询——查Point元素
查询字段的类型
|
|
构造box参数
box格式 [左下,然后右上]
|
|
Point注意导入的是geo下的
使用mongoRepository方式查询
|
|
使用mongoTemplate方式查询
|
|
使用BasicDBObject方式查询
|
|
空间查询——查Polygon元素
官网:The specified shape can be either a GeoJSON Polygon
查询字段的类型
|
|
构造框选参数
(不能用box了,查不出来,必须用Polygon/GeoJsonPolygon)
|
|
选择框属性的类型必须是GeonPolygon
使用mongoRepository方式查询
|
|
使用mongoTemplate方式查询
|
|
注意:mongoRepository只支持within的查询,要使用intersects的查询必须使用mongoTemplate,同时使用intersects查询时查询框必须是GeoJsonPolygon类
遇到的问题
空间查询点要素,范围框选太大查不出来 (已解决)
|
|
使用上面的测试数据, 运行下面的代码返回的结果为空
|
|
下面这个查得出来
|
|
做了下测试,发现Within对Polygon
类的支持比较好,虽然GeoJsonPolygon继承Polygon类,但是当范围很大的时候就是查不出来
还有一点要注意的是,在mongodb中空间查询使用的是$geoWithin
, 但是为什么在mongorepository中用的是within
呢?
mongoRepository
使用within
== 在mongodb
中使用$geoWithin
空间查询polygon,范围框选查不到所有包含在内的数据
|
|
经过多次对 $geoIntersects
的实验,发现几个现象:
1.经度的查询跨度必须小于180°
2.如果经度查询跨度大于180°,只会显示出数据库中polygon字段与查询范围相交
的记录
3.但是做了个测试经度范围在-170~140
竟然所有的都能查得出来,而且查出来的记录并不在范围内
查看官网的这句哈可以发现,当超过半个球的时候,他会查询一个互补几何,我猜测可能就是这块橙色区域,所以可以把所有的都查出来
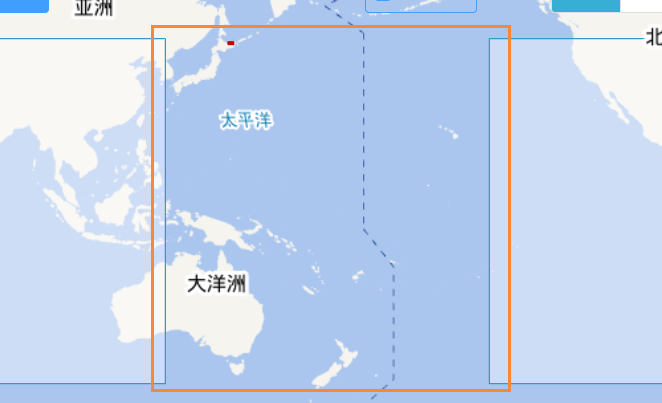
再做一个测试,框选一个范围,该范围经度跨度大于180°,且包含数据库中的记录下图红框。会发现一个都没查出来,这就可以证实了当经度跨度大于180的时候,他确实找的是红色的这块区域,也即
(-180°, 180°) - (leftLon, rightLon)
集合区域
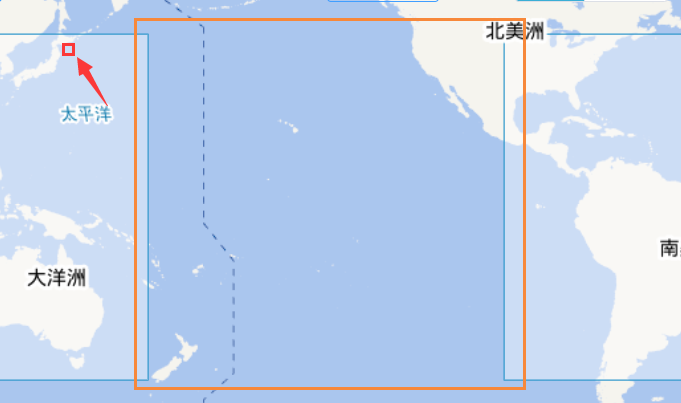
4.经度最大不能超过180°,最小不能少于180°,不然会报错 longitude/latitude is out of bounds
依据上面的现象完成了针对不同情况的空间查询
Service
|
|
Dao
|
|
MongoDB语法
|
|
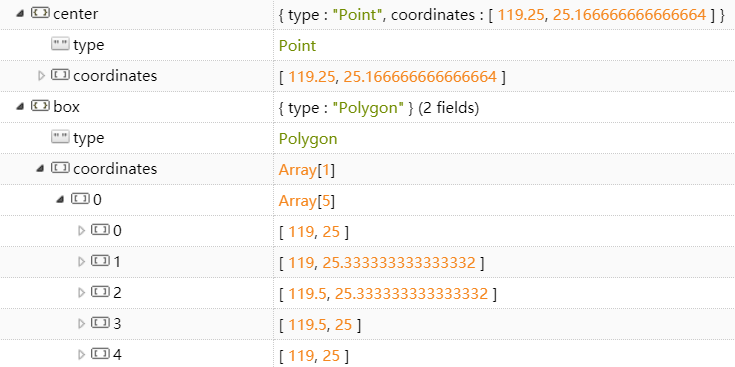
创建空间索引
创建索引
db.collection.createIndex(keys, options)
语法中 Key 值为你要创建的索引字段,1 为指定按升序创建索引,如果你想按降序来创建索引指定为 -1 即可
db.col.createIndex({"title":1})
创建空间索引
https://docs.mongodb.com/manual/core/2dsphere/
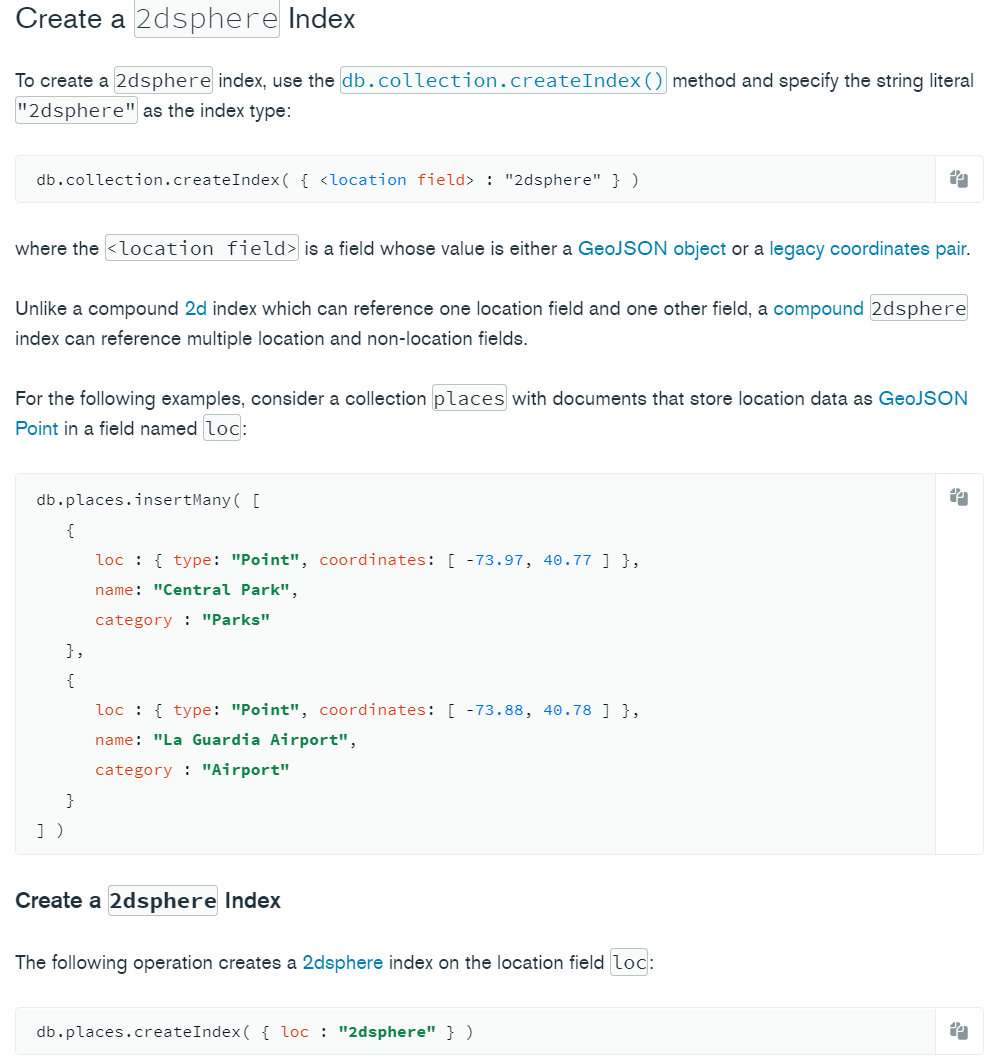
db.mapItem.createIndex( { center : "2dsphere" } )